Note
Go to the end to download the full example code.
e-ph matrix along a path in the BZ
This example shows how to use the GPATH.nc file produced by ABINIT to plot e-ph matrix elements along a path in the BZ.
import os
import abipy.data as abidata
from abipy.eph.gpath import GpathFile
Here we use one of the GPATH files shipped with abipy with the e-ph matrix g(k,q) with q along a path and k fixed. Replace the call to abidata.ref_file(“”) with the path to your GPATH.nc
================================= File Info =================================
Name: teph4zpr_9o_DS1_GPATH.nc
Directory: /home/runner/work/abipy/abipy/abipy/data/refs/teph4zpr
Size: 120.01 kB
Access Time: Wed Jan 29 13:41:39 2025
Modification Time: Wed Jan 29 13:35:24 2025
Change Time: Wed Jan 29 13:35:24 2025
================================= Structure =================================
Full Formula (Mg1 O1)
Reduced Formula: MgO
abc : 3.007126 3.007126 3.007126
angles: 60.000000 60.000000 60.000000
pbc : True True True
Sites (2)
# SP a b c
--- ---- --- --- ---
0 Mg 0 0 0
1 O 0.5 0.5 0.5
Abinit Spacegroup: spgid: 0, num_spatial_symmetries: 48, has_timerev: True, symmorphic: False
=========================== Electronic Bands (kq) ===========================
Number of electrons: 16.0, Fermi level: 6.729 (eV)
nsppol: 1, nkpt: 42, mband: 20, nspinor: 1, nspden: 1
{}
Direct gap:
Energy: 4.479 (eV)
Initial state: spin: 0, kpt: [+0.000, +0.000, +0.000], band: 7, eig: 4.490, occ: 0.000
Final state: spin: 0, kpt: [+0.000, +0.000, +0.000], band: 8, eig: 8.969, occ: 0.000
Fundamental gap:
Energy: 4.479 (eV)
Initial state: spin: 0, kpt: [+0.000, +0.000, +0.000], band: 7, eig: 4.490, occ: 0.000
Final state: spin: 0, kpt: [+0.000, +0.000, +0.000], band: 8, eig: 8.969, occ: 0.000
Bandwidth: 72.521 (eV)
Valence maximum located at kpt index 0:
spin: 0, kpt: [+0.000, +0.000, +0.000], band: 7, eig: 4.490, occ: 0.000
Conduction minimum located at kpt index 0:
spin: 0, kpt: [+0.000, +0.000, +0.000], band: 8, eig: 8.969, occ: 0.000
TIP: Use `--verbose` to print k-point coordinates with more digits
To plot the e-ph matrix elements as a function of q.
gpath_q.plot_g_qpath(band_range=None,
which_g="avg",
with_qexp=0,
scale=1,
gmax_mev=250,
ph_modes=None,
with_phbands=True,
with_ebands=False,
)
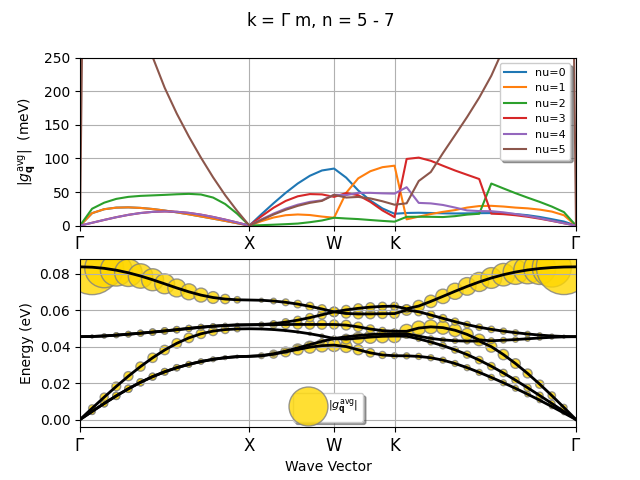
For the meaning of the different arguments, see the docstring
print(gpath_q.plot_g_qpath.__doc__)
Plot the averaged |g(k,q)| in meV units along the q-path.
Args:
band_range: Band range that will be averaged over (python convention).
If None all bands are considered.
which_g: "avg" to plot the symmetrized |g|, "raw" for unsymmetrized |g|. "all" for both.
with_qexp: Multiply |g(k, q)| by |q|^{with_qexp}.
scale: Scaling factor for the marker size used when with_phbands is True.
gmax_mev: Show results up to gmax in meV.
ph_modes: List of ph branch indices to show (start from 0). If None all modes are shown.
with_phbands: False if phonon bands should now be displayed.
with_ebands: False if electron bands should now be displayed.
ax_mat: List of |matplotlib-Axes| or None if a new figure should be created.
fontsize: fontsize for legends and titles
Keyword arguments controlling the display of the figure:
================ ====================================================
kwargs Meaning
================ ====================================================
title Title of the plot (Default: None).
show True to show the figure (default: True).
savefig "abc.png" or "abc.eps" to save the figure to a file.
size_kwargs Dictionary with options passed to fig.set_size_inches
e.g. size_kwargs=dict(w=3, h=4)
tight_layout True to call fig.tight_layout (default: False)
ax_grid True (False) to add (remove) grid from all axes in fig.
Default: None i.e. fig is left unchanged.
ax_annotate Add labels to subplots e.g. (a), (b).
Default: False
fig_close Close figure. Default: False.
plotly Try to convert mpl figure to plotly.
================ ====================================================
Here we read another file with the e-ph matrix g(k,q) with k along a path and q fixed.
================================= File Info =================================
Name: teph4zpr_9o_DS2_GPATH.nc
Directory: /home/runner/work/abipy/abipy/abipy/data/refs/teph4zpr
Size: 91.79 kB
Access Time: Wed Jan 29 13:41:40 2025
Modification Time: Wed Jan 29 13:35:24 2025
Change Time: Wed Jan 29 13:35:24 2025
================================= Structure =================================
Full Formula (Mg1 O1)
Reduced Formula: MgO
abc : 3.007126 3.007126 3.007126
angles: 60.000000 60.000000 60.000000
pbc : True True True
Sites (2)
# SP a b c
--- ---- --- --- ---
0 Mg 0 0 0
1 O 0.5 0.5 0.5
Abinit Spacegroup: spgid: 0, num_spatial_symmetries: 48, has_timerev: True, symmorphic: False
============================ Electronic Bands (k) ============================
Number of electrons: 16.0, Fermi level: 6.729 (eV)
nsppol: 1, nkpt: 42, mband: 20, nspinor: 1, nspden: 1
{}
Direct gap:
Energy: 4.479 (eV)
Initial state: spin: 0, kpt: [+0.000, +0.000, +0.000], band: 7, eig: 4.490, occ: 0.000
Final state: spin: 0, kpt: [+0.000, +0.000, +0.000], band: 8, eig: 8.969, occ: 0.000
Fundamental gap:
Energy: 4.479 (eV)
Initial state: spin: 0, kpt: [+0.000, +0.000, +0.000], band: 7, eig: 4.490, occ: 0.000
Final state: spin: 0, kpt: [+0.000, +0.000, +0.000], band: 8, eig: 8.969, occ: 0.000
Bandwidth: 72.521 (eV)
Valence maximum located at kpt index 0:
spin: 0, kpt: [+0.000, +0.000, +0.000], band: 7, eig: 4.490, occ: 0.000
Conduction minimum located at kpt index 0:
spin: 0, kpt: [+0.000, +0.000, +0.000], band: 8, eig: 8.969, occ: 0.000
TIP: Use `--verbose` to print k-point coordinates with more digits
To plot the e-ph matrix elements as a function of q.
gpath_k.plot_g_kpath(band_range=None,
which_g="avg",
scale=1,
gmax_mev=250,
ph_modes=None,
with_ebands=True,
)
![q = [0.110, 0.000, 0.000] m, n = 5 - 7](../_images/sphx_glr_plot_gpath_002.png)
For the meaning of the different arguments, see the docstring
print(gpath_k.plot_g_kpath.__doc__)
Plot the averaged |g(k,q)| in meV units along the k-path
Args:
band_range: Band range that will be averaged over (python convention).
which_g: "avg" to plot the symmetrized |g|, "raw" for unsymmetrized |g|."all" for both.
scale: Scaling factor for the marker size used when with_phbands is True.
gmax_mev: Show results up to gmax in meV.
ph_modes: List of ph branch indices to show (start from 0). If None all modes are show.
with_ebands: False if electron bands should now be displayed.
ax_mat: List of |matplotlib-Axes| or None if a new figure should be created.
fontsize: fontsize for legends and titles
Keyword arguments controlling the display of the figure:
================ ====================================================
kwargs Meaning
================ ====================================================
title Title of the plot (Default: None).
show True to show the figure (default: True).
savefig "abc.png" or "abc.eps" to save the figure to a file.
size_kwargs Dictionary with options passed to fig.set_size_inches
e.g. size_kwargs=dict(w=3, h=4)
tight_layout True to call fig.tight_layout (default: False)
ax_grid True (False) to add (remove) grid from all axes in fig.
Default: None i.e. fig is left unchanged.
ax_annotate Add labels to subplots e.g. (a), (b).
Default: False
fig_close Close figure. Default: False.
plotly Try to convert mpl figure to plotly.
================ ====================================================
Total running time of the script: (0 minutes 1.160 seconds)